Guide for building accessible websites in react
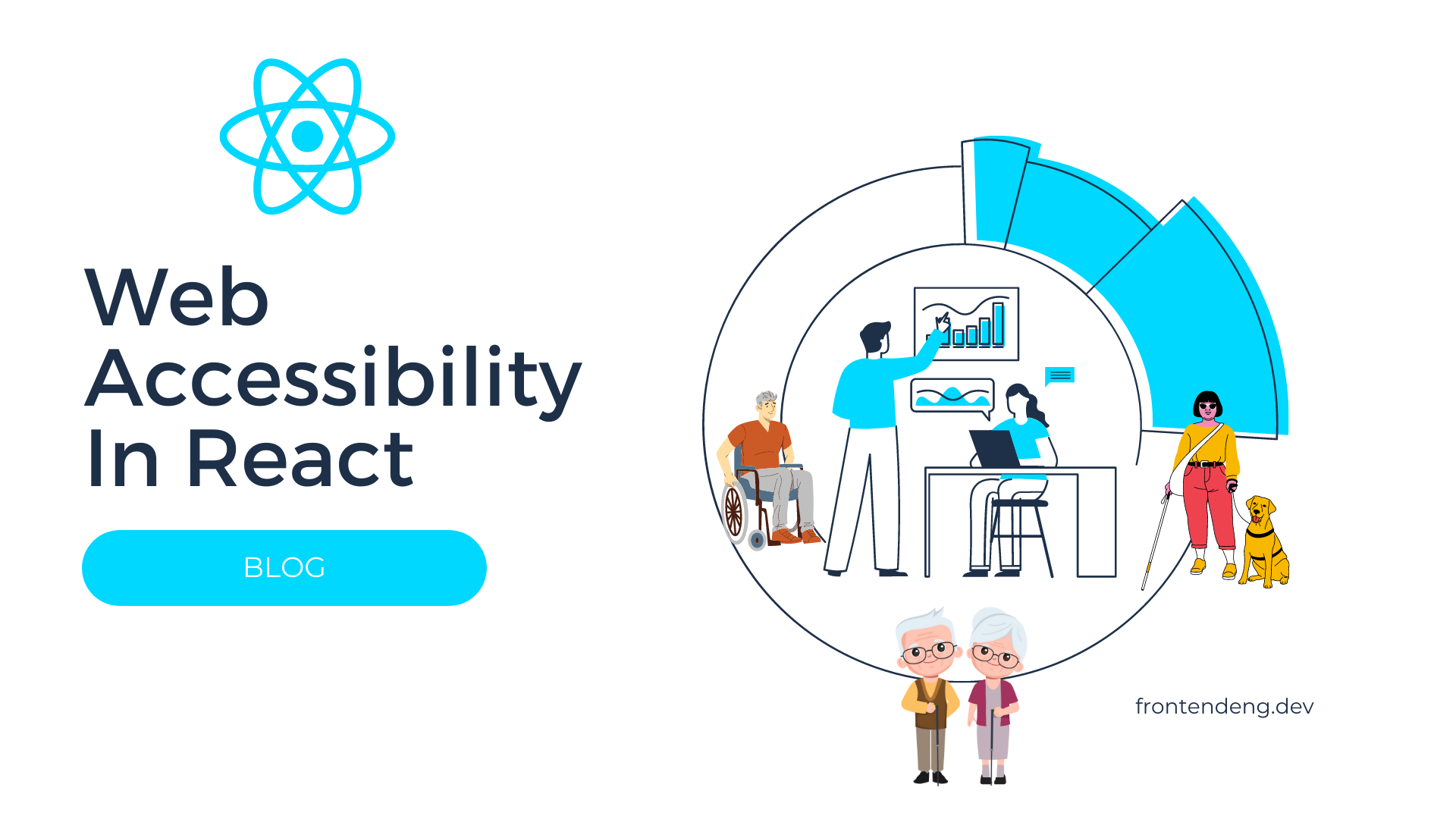
Web accessibility is the practice of designing and developing web content so that it can be accessed and used by people with disabilities. This includes people with visual impairments, hearing impairments, mobility impairments, cognitive impairments, and other disabilities.
Web-based applications are used by billions of people every day, and people rely on them to make their day-to-day life decisions. At that scale, a vast number of users may have some form of temporary or permanent disability that makes it harder for them to access the web application. It is important that a good engineer thinks about this right from the start and builds them in a way that everyone can use them well.
An accessible website is one that removes barriers and ensures that all users, regardless of their abilities, have equal access to its functionality. This includes people with temporary disabilities, such as a broken arm, and people with situational limitations, such as being unable to hear audio due to loud background noise. Assistive technology, such as screen readers and text-to-speech software, needs accessibility support to interpret web pages.
Table of contents
Understanding accessibility standards for web
Accessibility for web is often defined by standards and laws. In USA the primary law is ADA and the standard is WCAG. The current version of WCAG is 2.0. However accesibility needs are not merely limited to these two and there are business needs to why go beyond these as well.
Every front end developer must know at least the basics of these two.
WCAG Standard
Web Content Accessibility Guidelines (WCAG) is the most widely adopted accessibility standard for web content. It is a set of international guidelines that outline how to make web content accessible to people with disabilities. The WCAG are divided into three levels of conformance: Level A, Level AA, and Level AAA. Level A is the most basic level of conformance, while Level AAA is the most stringent.
Americans with Disabilities Act
The ADA for web refers to the application of the Americans with Disabilities Act (ADA) to websites and online content. The ADA requires that websites be accessible to individuals with disabilities, including people with visual impairments, hearing impairments, mobility impairments, and cognitive impairments.
WAI-ARIA
The Web Accessibility Initiative - Accessible Rich Internet Applications document contains techniques for building fully accessible JavaScript widgets. These are often known aria attributes and part of w3c defined standards of adding accessibility features in HTML.
Why accessibility for web matters ?
There are multiple benefits of an accessible design and implementation.
- More users get better experience. While each disability might be very tiny minority, together they do constitute much larger set of users.
- Disabilities often have much wider range and hence accessibility feature might make life better not just for disabled but also for those who are not considered disabled. For example, a person who might have forgotten to wear their glasses might benefit from use of color contrast and larger font sizes meant for the accessibility users.
- Products that cater to schools, small business etc. might have to comply with laws as well as business needs. For example a school for disabled students might want to use your software product. If the product is not accessible you lose the business.
- Ecommerce sites often see 1-2% higher conversions when their sites are accessible.
- Accessible websites are ranked highly by search engines. It improves your SEO score.
How to make a front-end accessible ?
The key principles of accessibility are the following:
- Using clear and concise language. Do not confuse the user with too many words or confusing languages. This helps everyone including your non disabled users.
- Using high-contrast colors and text sizes.
- Providing alternative text for images and videos.
- Making sure that all content can be navigated using a keyboard.
- Avoiding using flashing or blinking content.
- Testing the website with a variety of assistive technologies including screen readers.
How to make sure front-end is accessible and ADA compliant ?
Accessibility Audit
The front ends that you build must be regularly put through accessibility audit where you may use manual and auomated tools to understand how it works for accessibility users. Accessibility audit can figure out issues right from the start and help you resolve issues early.
There are a number of different tools that can be used to audit front-end code for accessibility. Some of these tools are manual, while others are automated. Manual tools require a human to review the code and identify any potential accessibility issues. Automated tools can scan the code for potential issues, but they may not be able to identify all of the issues.
AxeDev Tool
[AxeDev Tools for accessibility test] is very popular in industry for automated testing of your web application for accessibility issues. It helps developers find and fix accessibility issues in their code. It is a free and open-source tool that can be used with Chrome, Edge, and Firefox browsers.
WAVE
WAVE is also a very popular tool that can be used to detect accessibility issues with a given page. It can also detect issues like blicking and moving content. Wave-contrast is also a related tool that can help you test your web pages for different color contrasts.
WCAG 2.1 Checker
This is a free tool that can be used to check a website or web page against the Web Content Accessibility Guidelines (WCAG) 2.1.
How to chose the right tool ?
Some of the tools are highly automation oriented where as some are focused on manual testing. While manual testing is the only testing that can truly catch most accessibility issues, using some form of automatic testing in your developer workflow will help you can issues a lot early.
React and Accessibility
While accessibility is a framework independent concept, the framework you chose might make it easier for you to comply with accessibility requirements. React has extremely good support for accessibility and it has been used by thousands of websites specially catering to accessibility needs users.
React supports ARIA attributes in JSX
React uses JSX or TSX but you can very confidently continue using ARIA attributes in both. All aria attributes are supported by React. Also, while normal DOM attributes are camel cases for react, aria tags however can be used with their usual lisp-case.
For example:
React supports semantic HTML
Semantic HTML is the use of HTML markup to reinforce the semantics, or meaning, of the information in web pages and web applications rather than merely to define its presentation or look. Semantic HTML is processed by traditional web browsers as well as by many other user agents.
You can use all the normal semantic tags like header, footer, article, section etc. in React. Sometimes, JSX syntax forces you to return a single element and hence we often end up wrapping everything inside a single div, this break the semantic nature of the markup. React recognized this issue and provided us with the concept of fragment.
Accessible Forms in React
Forms are the most hard to get right part of accessible web applications. The key principles of making forms accessible are:
- Ensure form elements are named properly. Since forms natively provide various mechanisms for naming such as form content, labels, aria-label and many more you have to be careful in your choice.
- Ensure navigation is smooth even for keyboard users.
- Ensure errors are properly surfaced and user is correctly informed.
- Ensure focus is on right element at a given time.
React supports every feature that is natively supported by HTML. However, for focus React lets you directly work with the dom reference of the element.
Accessible Mouse Events in React
React components support a lot of mouse events. However, any mouse event needs to also have an equivalent keyboard focus and onBlur event. React components also support onFocus and onBlur events. For example see the below code that does not just rely on mouse events but also explicitly handles keyboard focus and blur events.
React development tools to assist in Accessibility
React has several tools that can help you think about accessibility right from start.
eslint-plugin-jsx-a11y
The eslint-plugin-jsx-a11y plugin for ESLint provides AST linting feedback regarding accessibility issues in your JSX. Many IDE’s allow you to integrate these findings directly into code analysis and source code windows.
aXe, aXe-core and react-axe
We had discussed the axe tools in earlier section, react has a package specifically for working with these tools.
Deque Systems offers aXe-core for automated and end-to-end accessibility tests of your applications. This module includes integrations for Selenium.
The Accessibility Engine or aXe, is an accessibility inspector browser extension built on aXe-core.
You can also use the @axe-core/react module to report these accessibility findings directly to the console while developing and debugging.
react-spectrum
React Spectrum is a large library of accessible React components that are based on the WAI-ARIA standards. It includes components for things like menus, dialogs, tables, and more.
a11y-react
A11y-react is a small library of utilities that can help you make your React code more accessible. It includes things like a function to generate alt text for images, and a function to check the contrast between text and background colors.
Accesibility Tree
While browsers internally represent markup as DOM tree, there is also an equivalent accessibility tree that is meant for cosumption of accessibility technologies such as screen readers.
Manual testing tools
Commonly Used Screen Readers
- NonVisual Desktop Access or NVDA is an open source Windows screen reader that is widely used.
- Job Access With Speech or JAWS, is a prolifically used screen reader on Windows.
- ChromeVox is an integrated screen reader on Chromebooks and is available as an extension for Google Chrome.
Your keyboard
Keyboard testing is perhaps the most simplest of testing. It is also worth noting that keyboard is not only used by people who might be disabled but also power users who use your tools very heavily. Sometimes keyboard is simply faster than the mouse.
Good keyboard navigation hence can benefit both your accessibility users and power users.
FAQS
What questions should I ask around accessibility to react fronend engineering job interview candiates
Ask candidates what they think about accessibility and what common problems they know around accessibility. Given them a simple problem and ask them to outline the accessibility problems first and then ask them about the possible solutions. Note that unless you are interviewing an accessibility specialist, candidate might not know the solutions upfront but must know the basics such as modeling components using forms helps you with accessibility.
I am a candidate interviewing for accessibility specific role, how should I prepare ?
If you are an react engineering preparing for an interview that is specifically about accessibility, you should familiarize yourself with WCAG standard and ARIA attributes exensively. Study all the ARIA-WCAG specific accessibility patterns here.
My company wants to build accessible applications, what is a good resources to get started ?
We recommend you start following WebAIM.
WebAIM stands for Web Accessibility in Mind. It is a non-profit organization dedicated to expanding the potential of the web for people with disabilities by providing knowledge, technical skills, tools, organizational leadership strategies, and vision that empower individuals and organizations to create and deliver accessible content.
WebAIM provides a variety of resources to help people make their websites and web content more accessible, including:
Accessibility tutorials: These tutorials provide step-by-step instructions on how to make your website or web content more accessible.
Accessibility resources: This section provides a variety of resources, such as articles, videos, and tools, on web accessibility.
Accessibility testing tools: WebAIM offers a number of accessibility testing tools that can help you identify and fix accessibility issues on your website or web page.
Accessibility certification: WebAIM offers a certification program for web developers and designers who want to demonstrate their knowledge of web accessibility.
Accessibility consulting: WebAIM offers consulting services to help organizations make their websites and web content more accessible.
Your company can start adopting their checklists and training programs to get started.
References :
https://hubpages.com/technology/why-web-accessibility-matters