What is useRef in react and how to use it correctly
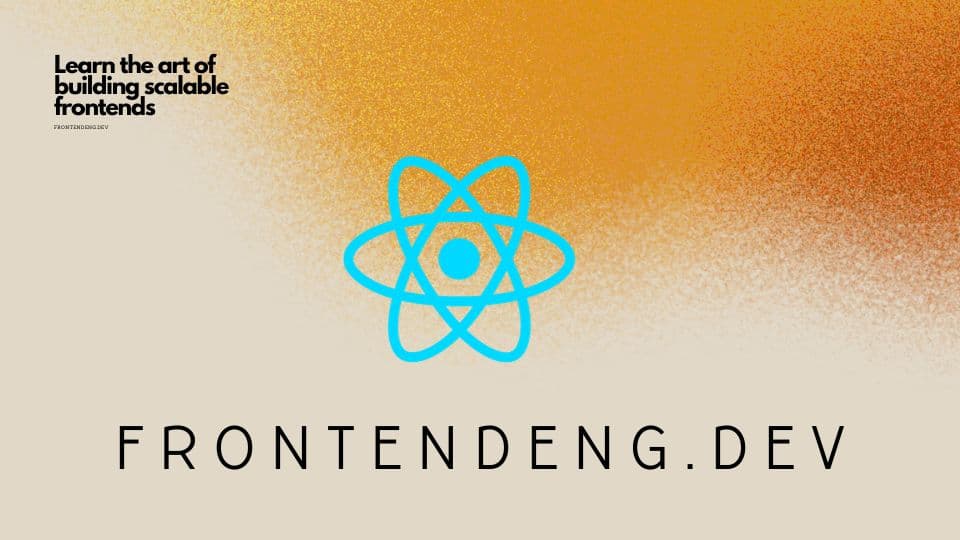
useRef is a React hook that allows you to obtain a reference to the underlying DOM object associated with a React component. It is particularly useful when you need to make direct changes to the DOM object without relying on other React primitives.
Table of content
When refs are needed
In most normal components you do not need a ref. Ideally usage of this hook should be avoided as much as possible as it makes the code harder to understand and changes to dom a bit more bug prone. But there might be some valid cases where this is needed. When you make changes to DOM using a ref, it bypasses the virtual DOM of react.
Some of the valid use cases where useRef is acceptable
Using third party libraries that don't play well with React
There are many useful libraries such as D3.js which you might want to use but they don't play well with react ecosystem. In such cases it might be sensible to use them to manipulate the DOM directly. It is a valid case but you should always remember that when these libraries make changes to the DOM, those changes will not be accessible to React's virtual DOM.
Some of the scenarios where such usage is normal is when you want to display charts, graphs drawn on an Canvas element.
Dealing with setTimeout and setInterval
If you are relying on these two methods in javascript you might want to use a useRef to cancel the setIntervals setTimeout invocations.
Creating variable that should not re-render the component when changes
You can use useRef to store a value that doesn't trigger re-renders when it changes, which is useful for some use cases like persisting data between renders.
Accessibility Focus Management
References is a common way to manage focus when you want to programmatically control where the keyboard focus should be even after the elment is re-renderd.
How to use useRef in React
Step 1: Use the hook
You can also provide an initial valye to the useRef hook.
Step 2: Assign the hook to the DOM
Step 3: Use the reference
Some examples
Using react with form elements
Conclusion
Avoid using useRef, but when you must use it, please make sure the use case is valid and there is no native react pattern to achieve what you are trying to achieve.
Please refer to the official documentation here.