Pages vs App directory in NextJS
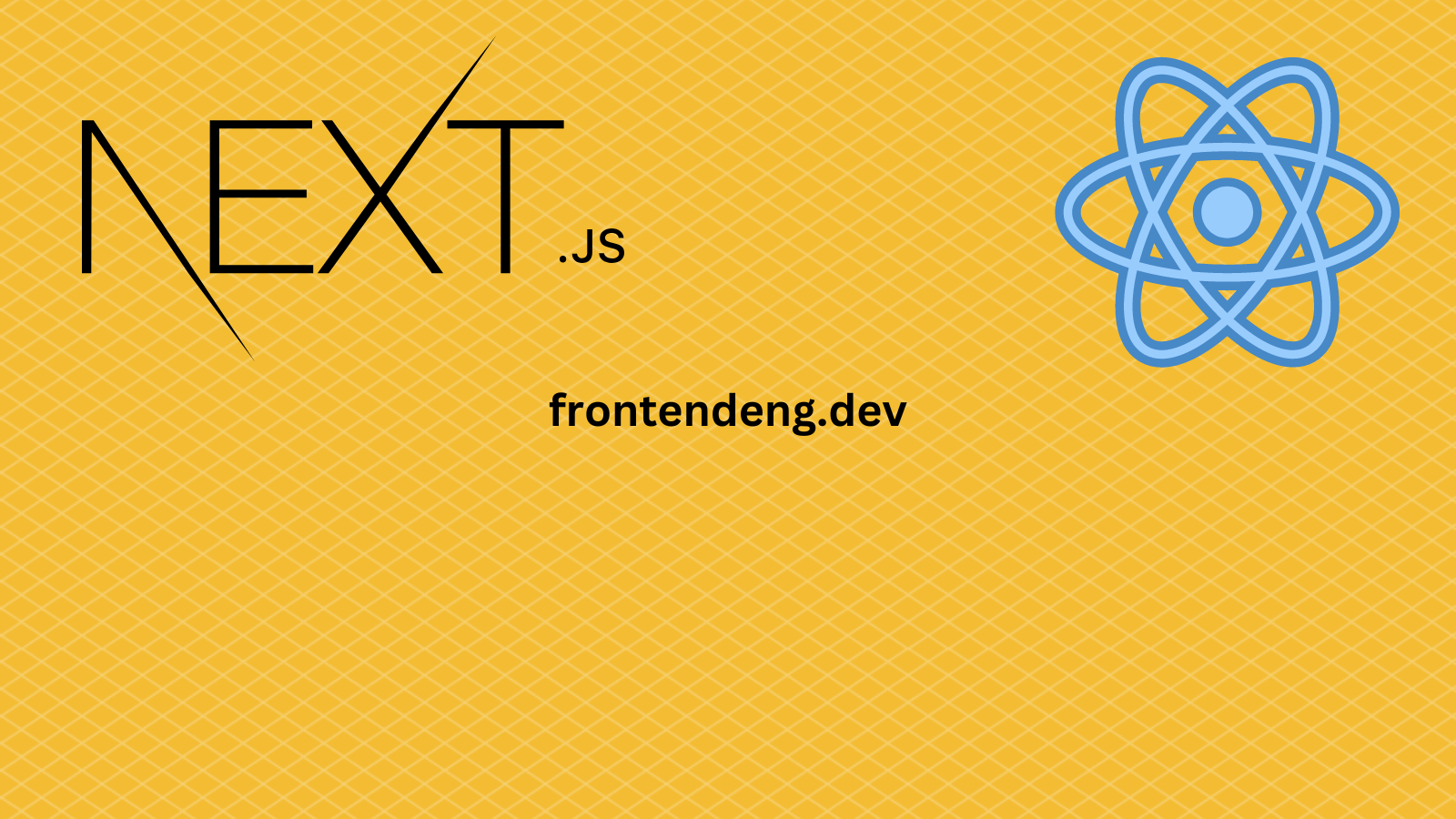
Nextjs introduced a new app router and app directory post version 13. While both app and pages directories serve similar purpose, the app router is expected to be better, more configurable and more performance focused and will eventually end up deprecating the the pages router. Developers should consider migrating to this new router and most certainly use app router if you are starting a new project.
Table of content
Pages directory
In nextjs you can put a component under pages to make it publicly accessible. For example pages/about.js
would get exposed as a /about
path for your webapp. This is how we build webapps using nextjs. If you have a small website with few pages you can continue to use this method to create your webapp.
Under pages, you have several ways to decide layout of your app, and you have access to nextjs's apis like getStaticProps
and getServerSideProps
. See official documentation here.
While nextjs has not made it clear if the future versions would remove this pages directory entirely, very likely it is going to happen some time in future. Which means if you are starting a new app that needs to be maintained, you should use app directory instead.
App directory
/app
was introduced in Nextjs version 13. It enforces server side rendering by default and makes use of React Server Components (RSC).
App is the new directory which has a special structure but servers identical purpose. In app every directory determines the path and the file that returns the component to be returned is always in page.js
. For example /app/about/page.js
is the file you need to create if you want to create a public path /about
.
The advantage of this approach is that it is safe to place arbitrary code under the app directory without worrying that it would get transformed into a publicly accessible url.
Secondly, a big difference between app and pages is the apis used to fetch data. We will see about that in next section.
App apis
The pages directory uses getServerSideProps and getStaticProps to fetch data for pages. Inside the app directory, these previous data fetching functions are replaced with a simpler API built on top of fetch
and async React Server Components.
In app directory you can simply use the standard react fetch and async apis without using the special apis like getServerSideProps. Note that nextjs will throw an error if you try to use the old apis.
If you want to see more examples you can always refer to the official documentation provided by nextjs that tells you how to migrate from pages to app.
Conclusion
Move the app directory in nextjs for your new projects and consider migrating. The biggest advantage is a very simple and intuitive api to fetch data instead of the old bloated hard to remember and argue about api.
References
1 - https://kristijan-pajtasev.medium.com/a-quick-dive-into-nextjs-app-folder-227ec88d5f3 2 - https://nextjs.org/docs/pages/building-your-application/routing/linking-and-navigating