A guide to conduct frontend engineering interviews for react roles
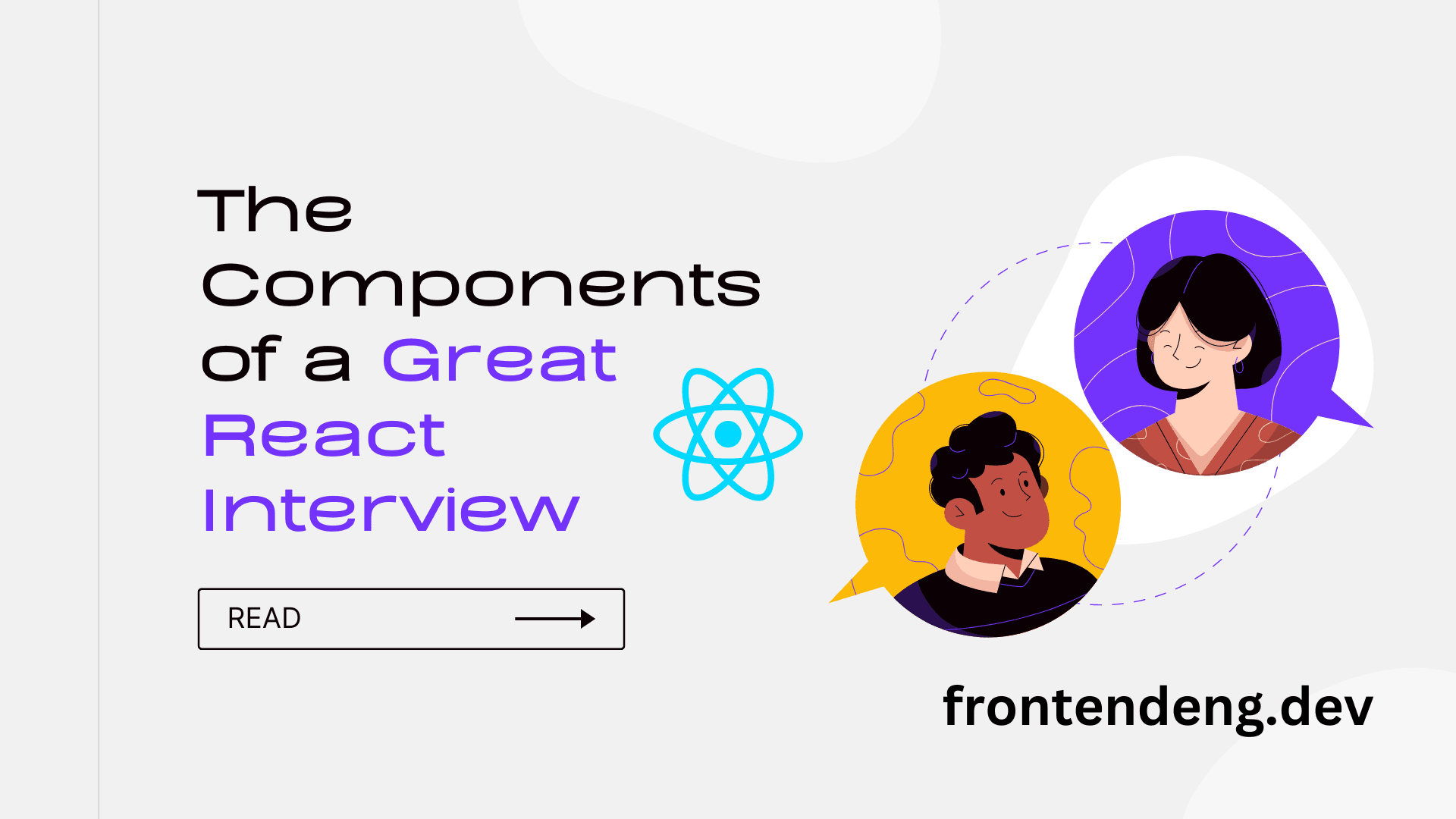
Interviewing candidates for frontend engineering roles is tough because of the constant changes in popular frameworks. When interviewing candidates for front end roles we recommend you try to evaluate candidates against.
- Knowledge of fundamental principles of front end engineering rather than knowledge of specific framework.
- Knowledge to day to day development methodologies rather than quirky specialized tasks.
- Check growth mindset and familiarity with trade offs.
- Verify candidate understands performance implications.
Table of contents
-
Five Dos of interviweing candidates for react roles
- Verify that candidate understands the need for React like library.
- Verify that the candidate can break down complex component into simpler component.
- Verify that the candidate can do component design
- Verify that the candidate understands common problems with React based front ends
- Ask candidate about certain react specific concepts
-
Ensure the interviwer has soft skills to conduct the interview
- Ensure the candidate knows which tool they will be tested on in advance
- Ensure that the candidate has the problem statement in writing during the interview
- Give candidate enough time to debug issues
- Do ask candidate why they chose a particular type of variable or why the split the component in a particular way
- Do ask candidate how they can improve their solution
Five Dos of interviweing candidates for react roles
We have picked 5 key dimensions along which you evaluate the candidate. We have also picked some sample questions that you may ask and criteria to evaluate the candidate on those lines.
Verify that candidate understands the need for React like library.
React is actually a library and not a framework. Angualar is a framework. But does the candidate understand why React exists or why it is widely used ? Has candidate ever wondered "why react?". Often this shows the caliber of the engineer you are going to work with. Candidate who asks deeper questions like these in day to day work can also spot other problems better. You can ask following questions to judge a candidate.
- What do you really like about React ?
- Given a choice, if you were to either pick React or just hand code HTML which one would you prefer ?
- If someone asks you why website like ours uses React instead of just plain vanilla HTML what will be your answer ?
These are open ended questions and there is no perfect answer here but we will look for candidate to demonstrate the following abilities.
- Candidate understands that React allows us to think about our UI in terms of Components and interactions between components. This simplifies development process, modularization and testability. You can ask the candidate to go deeper into any one of these topcis.
- Candidate understands the important loose coupling and testable code.
Verify that the candidate can break down complex component into simpler component.
The single biggest problem with react codebases these days is monolithic components. Even a simple button component can get very very complex pretty soon. A good developer can think about mitigating this problem right from the design phase.
One way to judge this is by asking questions like these :
- Given a simple table how would your break down this into a component hierarchy ?
- How would you implementing sorting functionality on this table ?
- How would you implement search box that filters the rows based on what user has typed ?
A good candidate weill tell you how this problem can be broken into multiple components. A DataTable component with DataTableHeader and DataTableRow components. DataSearchBox will have the search ability and they will communicate with each other using Redux or event mechanism.
Verify that the candidate can do component design
While components are designed to be independent units, they interact with rest of the UI using their API. A badly designed component API thus spoils everyones party.
Instead of asking cadidate to design something complex during interview take a very simple example but ask the candidate to provide detailed reasoning.
One of my favourite interview question is :
- Design a component DiceRoller. It is component that has one button and one label. If a user clicks that button the label will show a number between 1 and 6. The component will also show the last 6 outcomes. Rest of the UI will rely on this component for generating this outcome.
Candidate is expected to think here in terms of component API. This component will be used by others which could be some kind of games. Does the candidate think in terms of API ? Does the candidate design events to emit the outcome and updates to the history ? Does the candidate reports outcome and history in a single event or multiple ? Does the candidate think about race conditions where user clicks on the button several times very quickly ?
What data structure does the candidate chose to store last 6 outcomes ? What about last N outcomes ?
Verify that the candidate understands common problems with React based front ends
While react is a great technology it does have its problems. Ask the cadidate what they think these problems are. Again, here we do not expect right or wrong answers but it really tests if candidate has worked on real world problems.
Some question ideas are like these:
- In the codebase you have worked on what are some of the problems you have noticed ?
- Is there anything you do not like about react or working on react ?
- What is the most common cause of bugs in the react code you have seen ?
Some of the answers I have heard and liked are:
- Too much of reliance on third party libraries often leads to too much of bloat.
- Poor component design is often cause of really terrible code readability. Once a custom event or props are designed poorly and the component is used by dozen other components it is impossible to refactor it later.
- Poor use of useEffect and state management. It is important that we use useEffect as little as possible to avoid re-rendering of the component. Also, having fewer state management variables is also good for performance.
- Communication between components is where the react code gets really component. Imagine you have an "add to cart" button which adds an item to shopping cart and this needs to update the cart icon on the navigation bar. How exactly should we do this ? Redux solves this problem to some extent but it does make code lot more complex.
Ask candidate about certain react specific concepts
While I am completely against asking candidate anything about syntax and such, I would really want to make sure that candidate has written non trivial code in React. To this effect I expect the candidate to know at least basic React premitives.
Some example questions are:
- What is the purpose of useEffect hook in React ? What are some of the things we need to remember before using it?
- What is a Suspense component ?
- What are some limitations of React functional components compared to class components ?
I plan to write a much detailed post with all these sort of questions.
What you should look in the answers is not the fact that candidate can recall the details from memory but rather that the candidate is aware of these concepts and understand its design implications.
Five things you should not ask in a React interview
Don't test the candidate on syntax
Good front end engineers used solid tooling and work on several technologies. Expecting them to remember syntax out of memory is not fair and does not really test the caliber of the engineer. This is especially true if you are hiring a generic front end engineer and not someone who works only with react.
I recommend you give candidate skeleton code with all the basic things in place. I have seen interviewers expect candidates to even remember the import statements from memory.
Many candidates work with wide variety of react wrappers. Some write JS code some in JSX and some in TSX. All this leads to significant differences in syntax and how engineers things. These differences are irrelevant for the job for most companies.
What you want to test candidates on is not the syntactic knowlede but rather
- Component design
- State management
- API design
- Performance implications
- Problem solving
Don't expect candidate to do things they do not do on their job
Never ask candidates to do things like "create a react app in your IDE" or "setup redux to display a table".
Most engineers work on large codebases where "create app" isn't really something they do on day today basis. Their laptop might not even be setup for this sort of work.
Setting up Redux is int itself is a complicated step as it involves things like model design, setting up actions, reducers and so on. Expecting candidate to do things like these just waste of everyone's time.
Don't expect candidate to do UI polish unless that is the focus of the interview
Good engineers work on large applications where the themeing, css classes etc are designed in advance and are highly custom. Expecting the candidate to come up with CSS to make the UI look good is simply waste of everyone's time.
However, you can test candidate on their ability to think in terms of CSS classes. It is fair to expect them to apply classes to their markup with an intention of styling them but do not expect them to chose the styling itself.
However this does not apply if the job itself is about hiring a CSS expert who you expect to deliver pixel perfection.
Don't ask candidate to deal with forms
Forms are super important in web appliations but I would recommnd you avoid them completely in interviews or give candidate a skeleton code.
Most of the times interviewers design problems with forms which they pick up from internet. But in real world most applications deal with clever abstractions on top of usual low level forms API.
For example many companies use React hook form to simplify form handling. If candidates choses such type of library interviwers are cluess about what the candidate is writing. Sometimes things that you wish to test the candidate on gets solved trivially by such libraries.
A better alternative however is to give candidate a skeleton code and ask them to fill in the gaps you create to test them on specific things.
Don't ask candidate to solve algorithmic problems designed as React problems
Never mix algorithmic problems with React. It is simply bad interviewing. Inform the candidate beforehand if the coding interview wil lbe pure data structure and algorithm excercise or a React specific exercise.
I have seen interviewers come up with algorithmic problems and disguise them as React problems and then expect candidates to do things like recursion, depth first search and other things in React.
This fails to test candidate on both algorithms and React. Do not do this ever.
Ensure the interviwer has soft skills to conduct the interview
There should be some basic communication hygiene followed to conduct an interview.
Ensure the candidate knows which tool they will be tested on in advance
If you are going to make candidate use their own IDE of choice or if you are going to use something like codesandbox make sure candidate knows this in advance so they are prepared for the interview.
Ensure that the candidate has the problem statement in writing during the interview
Not all candidates are fluent in English and some might be hearing impaired or have problem understandig your accent. Make sure you provide a written statement.
Give candidate enough time to debug issues
Do not force the candidate to use specific debugging methods to debug any issue they encounter. See how they think.
Do ask candidate why they chose a particular type of variable or why the split the component in a particular way
This is to make sure candidate understands the tradeoffs in their design well.
Do ask candidate how they can improve their solution
In all cases ask candidate what they could have done better. Things I look for are.
- Candidate should be aware of any bugs if any.
- Candidate should be aware of any performance issues if any.
- Candidate should think about resuability and testability of their solution.
Conclusion
When hiring a front end engineer to work on your react app remember that you are hiring and engineer first and a react expert second. A good engineer works on any framework and can pick up any technology. For long term employment this is even crticial as these technologies evolve faster than we can keep track. Do not test the candidate on things that can be learned via rot learning or only through repitative work.
Interviewers must also do enough preparation for the interview and should not go into the interview unprepared. It is a waste of everyone's time and hurts the employers reputation.