How to disable cache for python http server
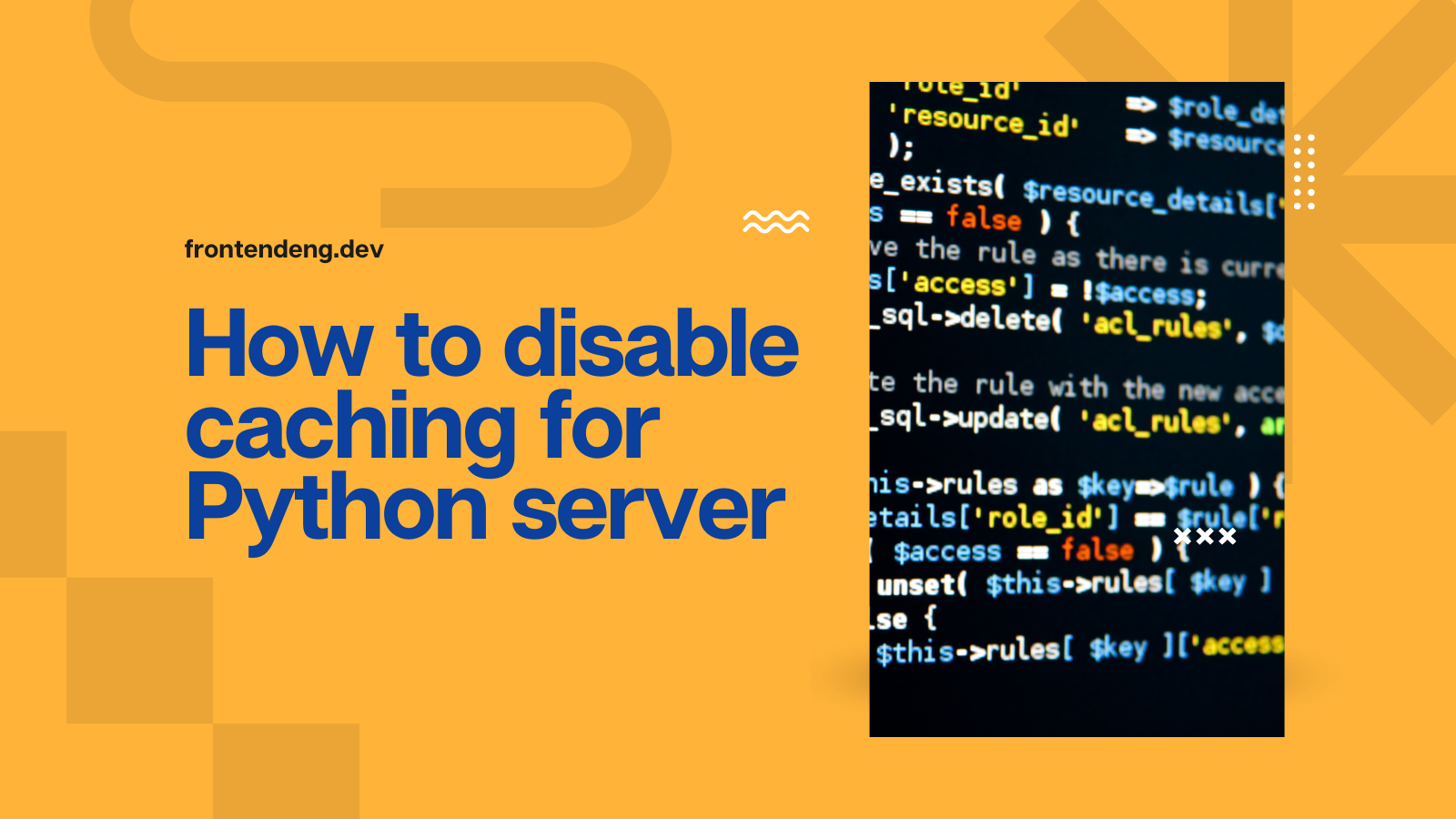
As a front end develoepr you will use wide variety of front end technologies. Sometimes you need a quick way to serve a directory using some kind of web server. Python3 is cross platform programming language that is available across all platforms and is often the easiest choice to spin off a web server locally.
Python local server
When building web pages with HTML, the ability to rapidly preview and test your changes is crucial. Python offers a remarkably straightforward method for launching a local server directly from your computer, sparing you the inconvenience of uploading files to a remote server each time you need to view updates.
For Python3
For Python2
In your browser's address bar, type http://localhost:8000/ (or replace 'index.html' with your HTML file's name if it's different):
http://localhost:8000/index.html
Disabling cache for this server
One problem with this server is that it caches everything. Which means when you make changes to your code, pressing refresh is not enough. One way to get around this is to press on the "disable cache" button in the chrome build tools and generally this is sufficient. But if you do not have access to chrome or can not do this for some reason you can also create a slightly different server usign python that does the job for you.
You can then run this sever as below where server.py is the name of the file.
But if you want to disable cache in chrome here is the option:
Conclusion
Are you ready to take charge of your HTML testing? Head over to your terminal, fire up that Python server, and let your creativity flow. If you encounter situations requiring more advanced features, don't hesitate to explore the world of Flask and Django.