Frontend Engineer's guide to GraphQL
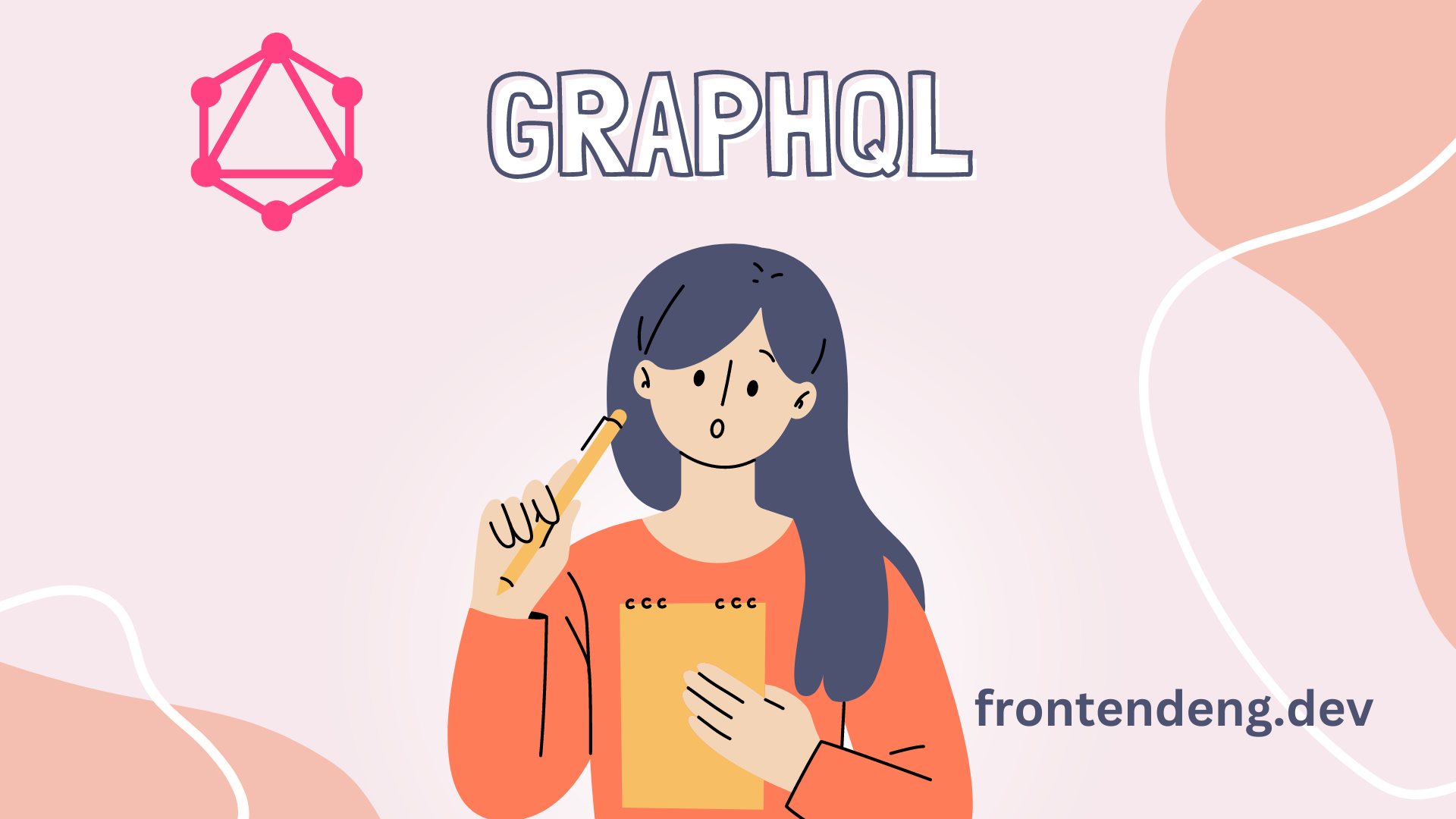
GraphQL is a query language and runtime for APIs that prioritizes giving clients exactly the data they request and no more. GraphQL is designed to make APIs fast, flexible, and developer-friendly by allowed developer to optimize the data that is fetched. GraphQL was created by Facebook in 2012 and is an open source project.
Table of contents
GraphQL in simple words
When it comes to fetching data from large databases SQL is the most popular querying language. Most query language has the following components.
- Which fields of data you want
- From which data source
- Which criteria it should satisfy
- How to order the data
For example SQL queries often look like
However with the advent of noSQL databases and more complex storage structures, queries become increasingly complex as they involve dozens of tables, several joins and complex ordering.
GraphQL solves this problem by letting you think of your data as a graph of different entities and then query an arbitrary portion of that graph. You dont have to worry about joins, tables, optimizing your query and so on.
For example when you want to fetch top 10 blog posts along with their authors information and top 5 comments the graphQL would look something like the following:
Doing the same using SQL would be incredibly complex and hard to read as well.
What should a front end engineer know about GraphQL ?
Unlike SQL which is primarily used by backend engineers, graphQL is incredibly popular among frontend engineers as it can be used from client code to query server code instead of REST API.
GraphQL often is sent to a HTTP endpoint and the endpoint returns with a JSON response. This is a better interface than traditional REST endpoints.
For example for the above example we will typically make around 20 REST calls. One to fetch top 10 posts and 10 to fetch top 5 comments of each of those posts.
Sometimes the backend engineers will create a new endpoint for you that can give you all the data you need in one endpoint but it means more work for everyone.
Every front end engineer thus needs to know at least basics of GraphQL as it is increasingly seen as a viable alternative for REST.
What GraphQL gets right.
GraphQL's flexibility leads to following main benefits
- Front ends can minimize the number of requests made to the server. This improves network performance.
- Front ends can fetch only precisely the data they need instead of requesting everything. This has performance benefits.
- There is no need for frontend enginers and backend engineers to collaborate to come up with new endpoints if GraphQL schema is fully made available. This improves development time significantly.
What GraphQL might get wrong
Overfetching
Like any technology GraphQL too has tradeoffs. The first one being is that its biggest strength its expressiveness is also its weakness. It means front end engineers now have too much power to query too much data. Also, lack of strict rules around schema means front end engineers might accidently fetch data they do not need.
Security
Security is another consideration. The backend might accidently expose all fields of an entity some of which might not be meant for clients to consume. For example user passwords.
Complexity
GraphQL is most certainly more complex than normal REST apis. Which meas the developers have to go through a learning curve. It also means possibility of more bugs.
Caching is hard
REST apis have well defined inputs which means caching is simpler for same inputs. GraphQL is not like that. The query for GraphQL is often sent as part of the body which is normally never used for caching. Caching GraphQL requires special configuration in your web servers.
Security risks
There is always a risk that a small misconfiguration on your server might expose more data to rest of the world than you want it to be exposed.
Maturity
GraphQL is relatively new technology compared to REST or the underlying SQL. Which means optimizations, performance improvements in GraphQL has still a long way to go. Several studies have shown that GraphQL services are computationally more expensive than SQL baser servers.
GraphQL in front end technologies
Static site generation
Static site generators like Gatsby use GraphQL to model its data. There are many other static site generators which can optionally use GraphQL.
Apollo Client
Apollo Client is an alternative to Redux. It provies a GraphQL interface for both client and server states.
Apollo Client is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL. Use it to fetch, cache, and modify application data, all while automatically updating your UI. Source
If as a frontend engineer you ever end up using GraphQL chances are you will end up using Apollo.
Headless CMS and GraphQL
Nearly all major headless CMS like Contentful, Strapi, Sanity etc. either support GraphQL by default or as an addon. GraphQL is a more natural choice for headless CMS as their schema can keep on evolving and change frequently.
Popular public APIs that use GraphQL
Here is a very large list of all well known public apis that use graphql as interface.
Some notable names are:
- Github
- Yelp
- Shopify
- Google directions API
Examples of GraphQL
Querying for a single field:
Querying for multiple fields:
Querying for a nested field:
This query will fetch the name of the hero, the names of their friends, and the names of their friends' friends from the database.
Querying for a field with arguments:
Querying for a fragment:
This query uses a fragment to define the fields that should be fetched for the hero. The fragment is then used to fetch the hero and their friends.
GraphQL query with sorting and ordering:
his query will fetch the first 10 posts from the database, ordered by the CREATED_AT field in descending order. The orderBy argument specifies the field that should be used to sort the results, and the direction argument specifies the order in which the results should be sorted.
In this example, the CREATED_AT field is used to sort the results, and the direction is set to DESC, which means that the results will be sorted in descending order. This means that the first post in the results will be the most recently created post.
The first argument specifies the number of results that should be returned. In this example, the first argument is set to 10, which means that the query will return the first 10 posts from the database.
Querying GraphQL from an React Component using Apollo Client
This code uses the ApolloProvider component to wrap the app. The ApolloProvider component takes a client as an argument, and it makes the client available to all of the components in the app.
The useQuery hook is used to fetch the posts from the GraphQL server. The useQuery hook takes a query as an argument, and it returns a state object with the loading, error, and data properties.
The loading property is true when the query is loading, and it is false when the query has finished loading. The error property is an error object if the query failed, and it is null if the query succeeded. The data property is the data that was returned by the query.
The App component uses the useQuery hook to fetch the posts from the GraphQL server. The App component then renders a list of the posts.
Conclusion
GraphQL knowledge is an important skill for any front-end developer to pick up. It is relatively simple laguage to learn and very intuitive in terms of interfaces. Its adoption is one rise and is only going to be more popular in future.