Why learn typescript ?
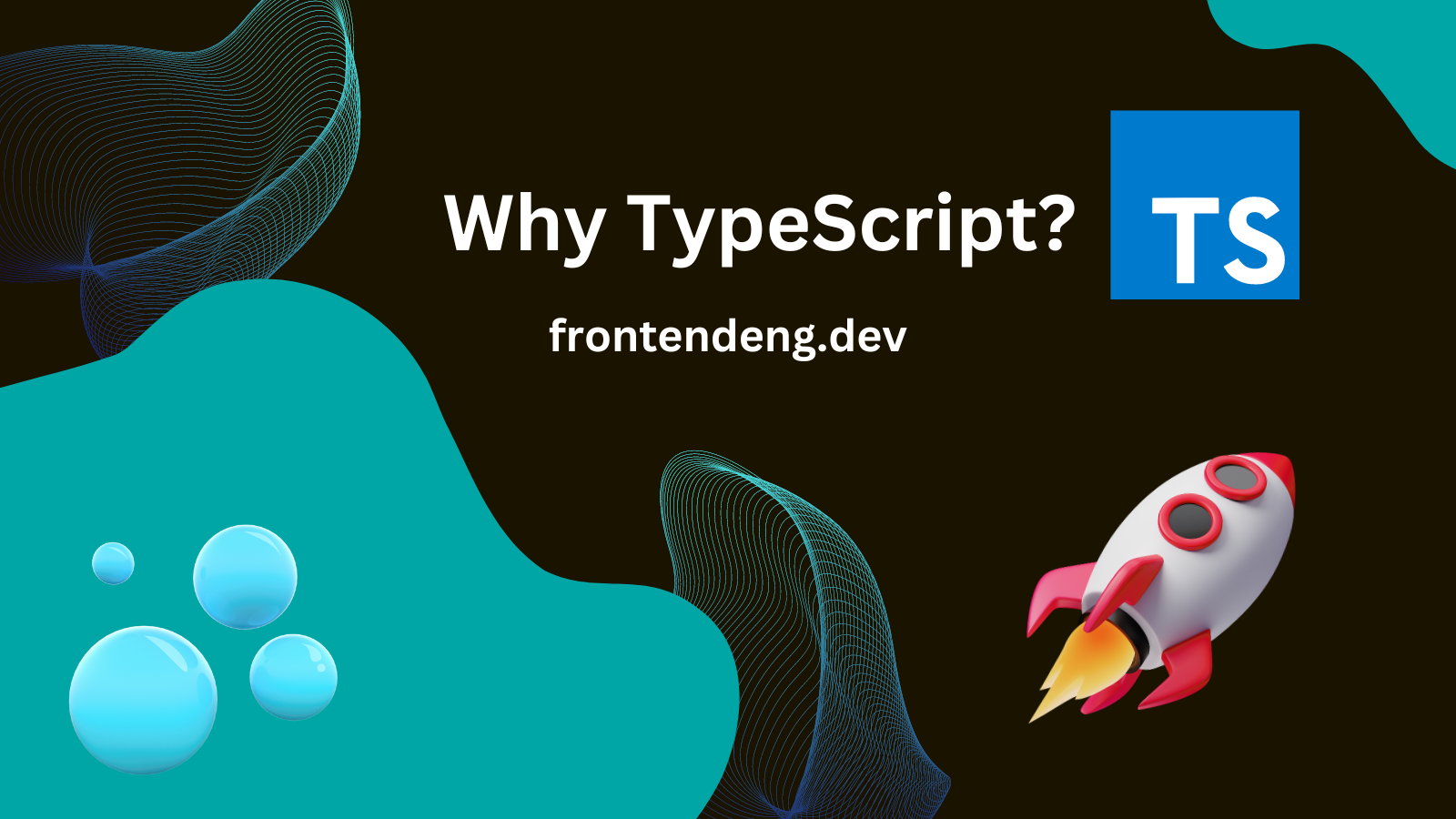
TypeScript, developed by Microsoft and released as open-source in 2012, was created to address the shortcomings of JavaScript for large-scale application development. While JavaScript remains the standard scripting language for web browsers, its limitations prompted the creation of several alternatives. These included Adobe Flash, Google's Dart, and languages like CoffeeScript. TypeScript has emerged as a dominant choice, offering improved tooling, type safety, and scalability, making it one of the most popular languages alongside JavaScript.
Table of contents
Typescript does not replace JavaScript
It is worth noting that TypeScript is not aimed at making JavaScript obsolete. TypeScript is a facade around JavaScript which means when you write TypeScript code it gets transpiled (convereted) to JavaScript which eventually runs on the browser. As a JavaScript developer you can always chose not to use Typescript at all. With modern JavaScript standards, a lot of Typescript like features are already part of JavaScript's core standard and hence modern JavaScript code is remarkably better than the JavaScript of 2012.
However, type checking is the most important feature of Typescript and it is unlikely to come to JavaScript anytime soon. Which means TypeScript will not become irrelevant like jQuery became irrelevant with time.
TypeScript translates into JavaScript
Browsers or nodejs can not execute TypeScript, Typescript compiler translates the TypeScript code into JavaScript. This process is called transpiliation. Typescript transpiler is extremely powerful and optimizes the JavaScript code as much as possible. The performance impact of using TypeScript is nearly zero as human programmers are unlikely to be that efficient in writing JavaScript code.
Once you have installed typescript compiler you can execute any arbitrary typescript code. Write your typescript code and store in the .ts file. Then execute the following code
This command takes typescript as input and converts it into a JavaScript .js file which you can execute using following command.
TypeScript features that make it a better language than JavaScript
Type Safety: The most important feature.
A lot of research shows that most of the bugs in non statically typed languages such as JavaScript or PHP are due to the lack of static typing. If static type checking existing in these langauges the programmers will have to write some extra code but at the same time, it would make their code much more robust.
TypeScript lets you define types for variables, functions, and objects. This is like putting labels on your data, so both you and the compiler understand how everything should fit together.
Consider this JavaScript code :
When you write such methods it can take any objects as arguments. For someone who is reading this code it is not clear whether price is a positive number, integer, float or something else. Same with the quantity. However in real world it is very likely that price would be a float and quantity will an integer and both will be positive numbers.
Same code can be written in TypeScript as follows:
Here both arguments are being specified as number and will not accept any other arguments when you are using it in your code. In TypeScript you can always define your own custom types as well such as below:
Maintainability and Scalability
TypeScript brings a lot of concepts from other successful programming languages like Java, C++ and Python. While JavaScript also supports concepts like inheritance it does it through more unusual and bug prone methods like prototypal inheritance. TypeScript on other hand it much easier to read and understand with its more standard class and interface based code.
TypeScript is much more generative AI friendly that JavaScript
Have you ever wondered when you type something in your IDE and when you type "obj." the IDE suggests you all the available methods automatically ? IDE is able to do that using wide variety of techniques. One is reflection, where it dynamically figures out what methods are actually available on that object. However, in JavaScript objects can be modified anytime sometimes dynamically during runtime, hence IDE can not figure all this out easily and make code recommendations.
TypeScript being statically typed, is much easier to be argued about and IDE finds it much easier to suggest your methdods and arguments etc.
Modern era belongs to AI and IDEs are increasingly using AI to suggest you code. Typed languages perform much better under such generative AI tools and can guess exactly what code you are writing.
TypeScript thus is more useful in era of AI.
Conclusion
Overall, TypeScript provides a robust foundation for building maintainable, scalable, and error-resistant applications that leverage AI-generated code. When the benefits outweigh the potential overhead, it's a compelling choice.