What is the difference between const, let and var in Javascript?
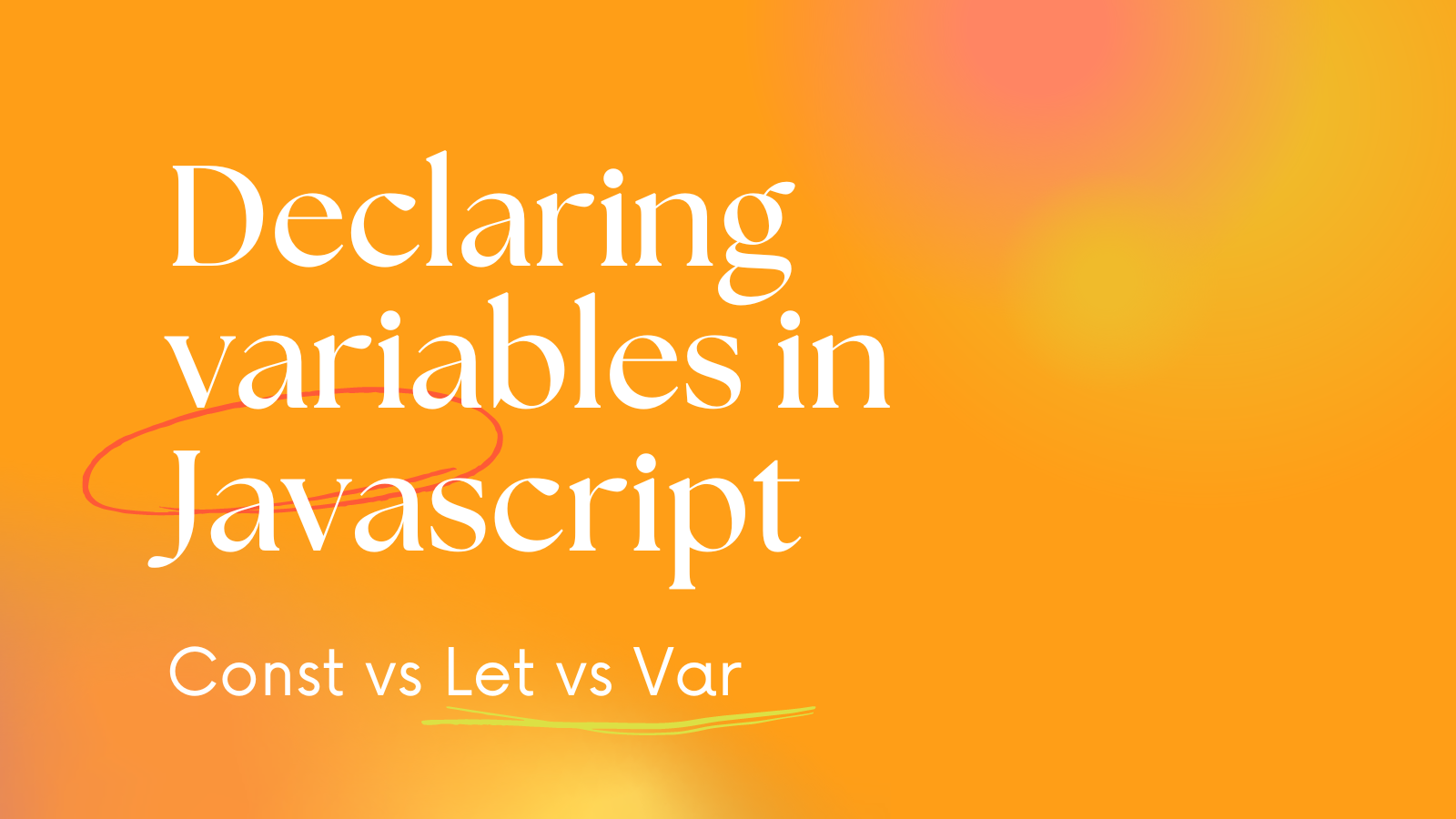
Declaring variables in Javascript.
In modern ES6 Javascript there are three modifiers to declare variables. These are let, const and var. var is most certainly a discouraged way to define a variable and exists solely for backward compatibility.
Var
You can declare variables using var if you want them to have function scope. Which means they are accessible anywhere inside the function.
Example:
If you ever use "var" outside the fuction is is basically a global variable. If you define a variable without ANY modifier then it is by default "var".
Avoid var completely. Always use let or const.
const
You should always declare your variables using const wherever possible. Once you assign a variable declared as const, its value van not be modified.
It is worth noting that you can not assign a new value to the const variable but that does not mean you can not modify the properties of the object stored in that variable.
Why const is better than let or var
One big source of bugs in any code is a programmer who is making wrong assumptions. If a variable is not a constant, a programmer might make an assumption that it gets a certain value and then write their code. Such mistakes are very expensive to catch because depending on the assumption it might not get caught until the bug reaches production.
However, with const the programmer can not make any assumption about its value and can think of it as an unchanging value.
This leads to safer code. Another advantage is that some Javascript optimizers can modify your code better if they detect it to be a constant.
Let
Another most popular way to define variables is by using let. It is very similar to var except that the scope of the variable is limited to "block" instead of function. A block is something that is between a set of curly brackets. {}.
Block-scoping: In the loop example, i is only needed within the for loop's block. let ensures it doesn't pollute the surrounding scope.
Mutability: In the score example, currentScore is intended to be updated. let allows for these necessary value changes.
Conclusion
We discussed the different ways to declare variables in ES6 JavaScript: let, const, and the older var. We focused on const, emphasizing its use for values that should remain unchanged, with examples like constants and configuration settings. We then explored let, highlighting its block-scoping and mutability, which make it ideal for variables needing updates or those used within specific code blocks.