What is prop drilling in React
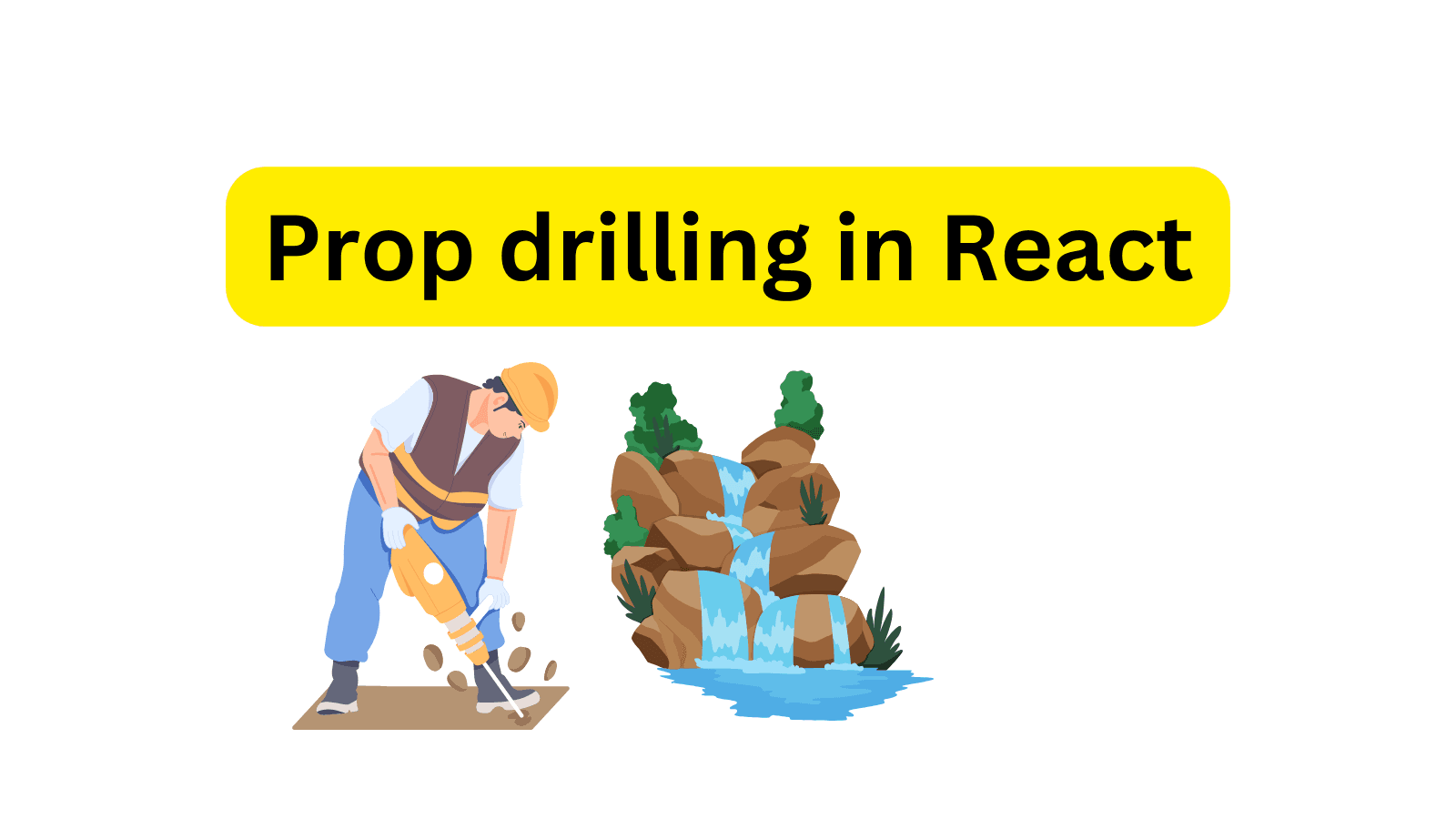
What is prop drilling in React ?
In React, components are often nested within each other, forming a tree-like structure. Data (state or values) typically resides at a higher level in this tree. Prop drilling refers to the process of passing data from a parent component down through multiple layers of intermediary components to reach a deeply nested child component that actually needs the data.
Example
In this example, the theme value is passed down from ParentComponent to Toolbar and then finally to Button, even though Toolbar doesn't directly use the theme value.
What are the alternatives ?
As one can see, when you have a very deep prop hierarchy, it might be complicated to pass many objects around. Sometimes, intermediate components do not need the object at all and still end up having it as a prop just because some child might need it.
This makes code harder to read and reason about, and also future refactoring becomes complex.
One of the alternatives is to use third party libraries like Redux.
Another approach is to use something called "Context" provided by React's useContext
hook. This is fairly complex and beyond the scope of this article to discuss.
When to use prop drilling
At its heart, React is designed around the idea of components passing data down to their children through "props" (properties). This direct parent-to-child communication is known as prop drilling. It's the most straightforward way to manage data flow in React. The relationship between components is clear and easy to follow, making your codebase more maintainable, especially for smaller projects.
Prop drilling is not an external library or pattern; it's fundamental to how React operates. This means you're not relying on any third-party tools that could change or become unsupported in the future. In many cases, prop drilling is efficient. React's Virtual DOM helps minimize unnecessary re-renders, and optimizations like memo or PureComponent can further improve performance. If you're using TypeScript with React, prop types can be strictly defined, providing excellent type safety and reducing the chance of errors.
References
Comprehensive guide to inter component communication in React React JS official docs