Why is key property important in react when displaying lists
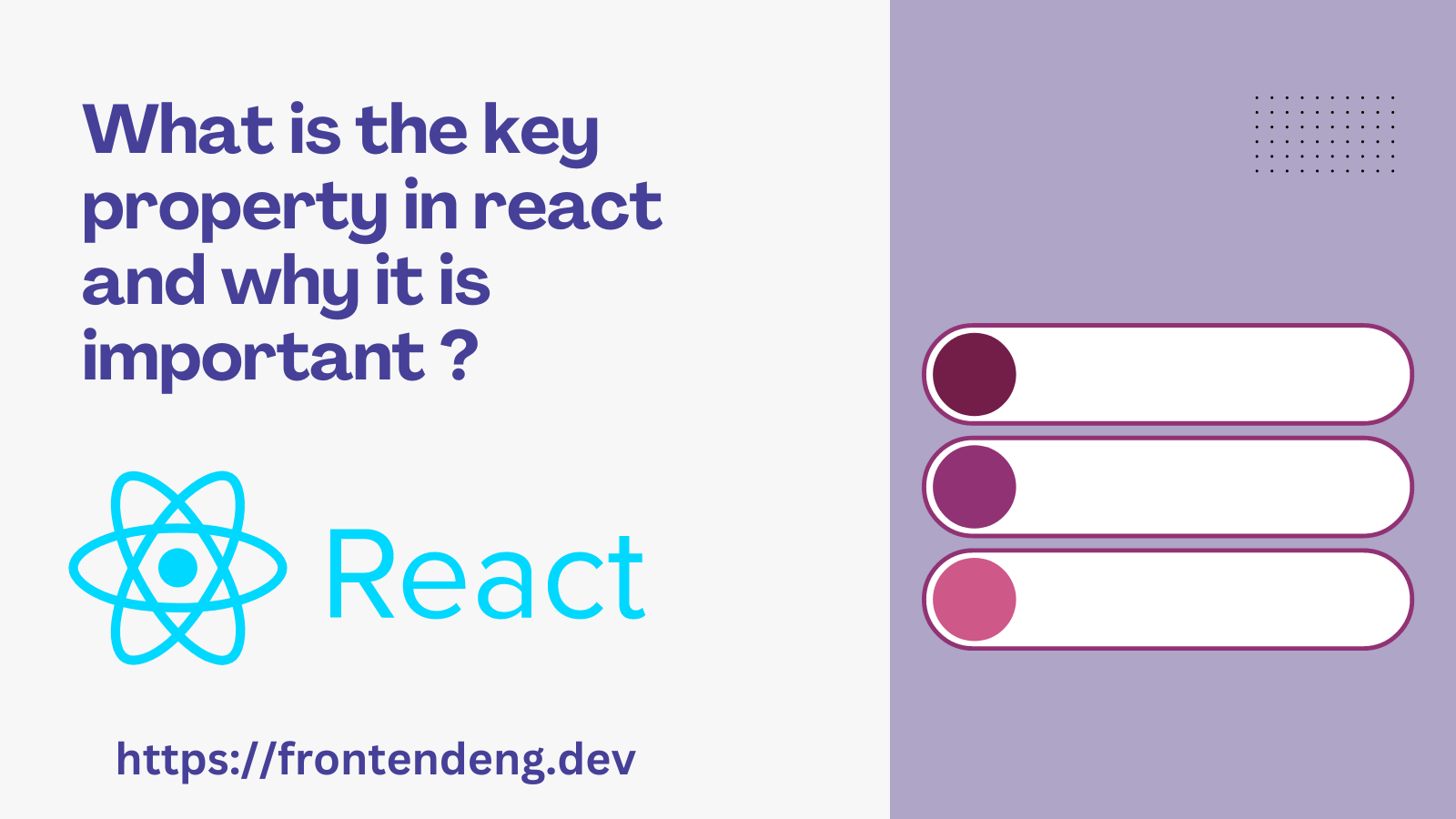
Each child in a list should have a unique "key" prop. Keys tell React which array item each component corresponds to, so that it can match them up later. This becomes important if your array items can move (e.g. due to sorting), get inserted, or get deleted. A well-chosen key helps React infer what exactly has happened, and make the correct updates to the DOM tree.
You can read about this in official documentation here.
Table of contents
- Rendering Lists in React
- What happens if we do not specify key property in a list ?
- How to generate the keys
- Interview questions about React Keys
- Asking interview questions around React State.
Rendering Lists in React
Lists are a well known thing in HTML. Everyone has used lists some time in their life.
But in React when we deal with react, we are not refering to the markup but rather how a markup is rendered. For example we might render a list as list of divs.
The above code is a static list and simple. But in reality lists are often dynamic and maintained as state.
In this code however the list may change and everytime it changes the entire list needs to be rendered again. This would be very problematic hence react likes to update only those items in the list that changed and maintains an internal mapping between the list data structure and the rendered elements.
To do this it need some unique identifier for the items in the list. This is often represented as "key"
What happens if we do not specify key property in a list ?
Imagine that files on your desktop didn’t have names. Instead, you’d refer to them by their order — the first file, the second file, and so on. You could get used to it, but once you delete a file, it would get confusing. The second file would become the first file, the third file would be the second file, and so on.
Keys tell React which array item each component corresponds to, so that it can match them up later. This becomes important if your array items can move (e.g. due to sorting), get inserted, or get deleted. A well-chosen key helps React infer what exactly has happened, and make the correct updates to the DOM tree. Read up official react documentation.
But if the key is not specified then react can not map the array items to the components, it by default relies on array index to do this. But if your array gets changed through sorting, this will mess up the entire list.
How to generate the keys
Ideally key property of the component should map to some unique attribute of the data it is representing. If it does not then you can generate a random ID for the data as unique property using libraries like UUID.
However never generate a random number on the fly because it will force React to rerender the elements every time the number is generated.
For example never do the following:
Interview questions about React Keys
This is easily the most common react interview question that gets asked and since it gets asked everyone knows the official answer. But it is important to show that besides knowing the official answer you understand the concept well. Always answer it in your own words.
For example:
It is very common pattern in react to use some data to render a list of components. Lists however change and these changes in most cases are sorting, deletion of specific element, addition of a new element and so on. However, for reach this is a change to entire list so it is forced to re-render all the items as if the entire list had changed. This is terrible for the performance. So react likes to map each React component to the actual data element in the list and accordingly instead of re-renderign entire list it likes to render only those elements in the list that changed. Key is a way react maps the react component to the element of the list. Without key, react defaults to the array index, which means if you sort, rearrange the list, all your components will get into a weird state.
Asking interview questions around React State.
If you are conducting react interviews I recommend reading our other post that gives you more detailed guide.
I do recommend asking each candidate a bit about react keys. You can either ask them directly or via going through their code.
One good way to ask if the candidate truly understand it is by asking the candidate:
- What happens if we do not use key at all ?
- What happens if we use a different property of data as key ?
- What if there is not unique property for the data ? What will you do ?